How to save entered values in Bash
Use a file and the declare command to ask information only once
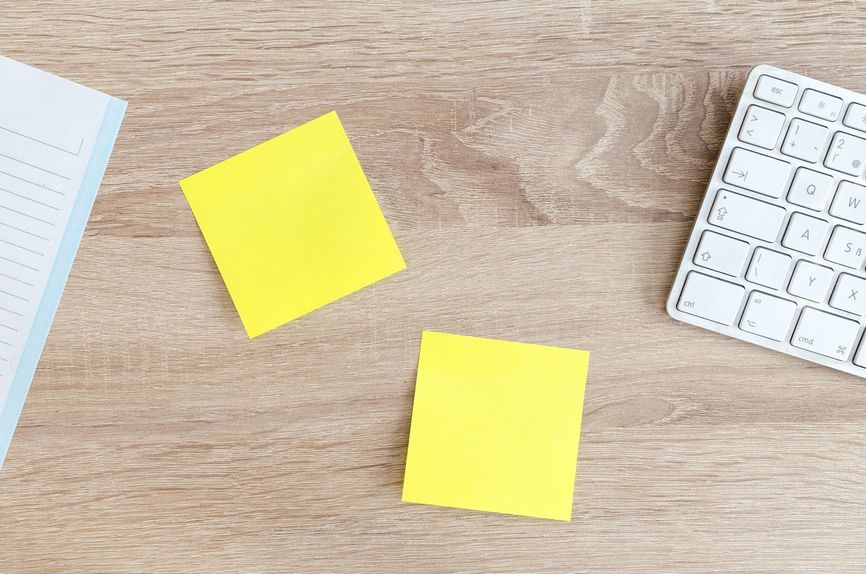
Input values
I use a dotfiles folder that sets up my development environment with all the tools and config I need for my work. One thing it does is it sets up a gitconfig file which is required to use git.
It needs a name and an email, and it has a trivial structure:
[user]
name = ...
email = ...
As I don't want to hardcode them the install script asks for these values:
read -p "Git username: " GIT_NAME
read -p "Git email: " GIT_EMAIL
When I enter these data, the script can go on and initialize the gitconfig.
The problem is, when I run it again it asks for the same info again. While it could parse the gitconfig, I wanted a solution that works with all sorts of other
services. So it should save the GIT_NAME
and the GIT_EMAIL
values somewhere and reuse them the next time the script runs.
declare
Bash has a function that prints the command that initializes a variable to its current value by outputting a declare
command:
declare -p GIT_NAME GIT_EMAIL
This prints:
declare -- GIT_NAME="...name"
declare -- GIT_EMAIL="...email"
Note: The --
means the type of the variable is not defined (string).
These commands restore the GIT_NAME
and the GIT_EMAIL
variables with their entered values.
Putting it together
With this, a simple solution is possible. It has three parts.
First, if a file with the saved variables exists, load it:
if [ -f "git/.saved_variables" ];
then
. git/.saved_variables
fi
Then check if the required values are present, and if not, prompt for them:
if [[ -z "$GIT_NAME" ]];
then
read -p "Git username: " GIT_NAME
fi
if [[ -z "$GIT_EMAIL" ]];
then
read -p "Git email: " GIT_EMAIL
fi
And finally, save the current values to the file:
declare -p GIT_NAME GIT_EMAIL > git/.saved_variables
This way, the script only asks for info the first time it runs, then the next time everything is automated.