GraphQL tip: Only make fields required if they are present for all users
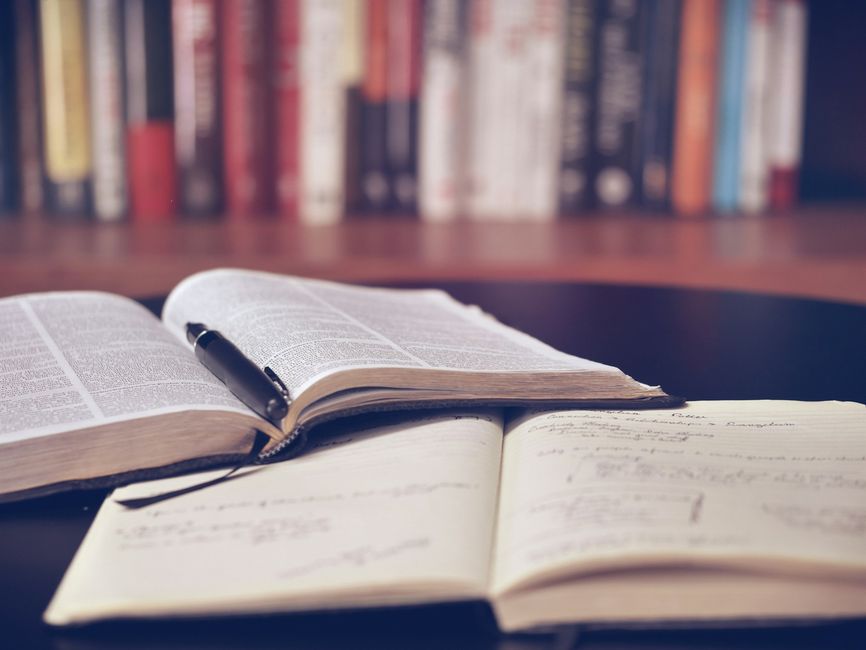
GraphQL tip: Only make fields required if they are present for all users
In GraphQL it's possible to mark a field as required with the !
. For example, a User
always have a Project
according to this schema:
type User {
name: String!
email: String!
project: Project!
}
Marking fields required is a powerful tool in the GraphQL toolbox as clients won't need handle the case when the field is null. But it can also lead to a cascading null effect.
For example, let's say the Project has a client
field that also can't be null:
type User {
name: String!
email: String!
project: Project!
tickets: [Ticket!]!
}
type Project {
name: String!
description: String!
client: String!
}
// + Ticket
Let's say the Project.client
is considered sensitive information and will be removed for non-admin users.
Then a query that gets the User
, its Project
, and and then the client
field will fail to return any data:
query {
user(id: "user1") {
name
email
project {
name
description
client
}
tickets: {
id
}
}
}
The result:
{
"data": null,
"errors": ...
}
Because of this, be mindful which fields you mark as required. If a field can be null for some users during normal operations, such as when you have different access levels, it should be optional. A field should be marked as mandatory only when it returns a value in every case.