AWS CDK best practice: include the region in the stack's ID
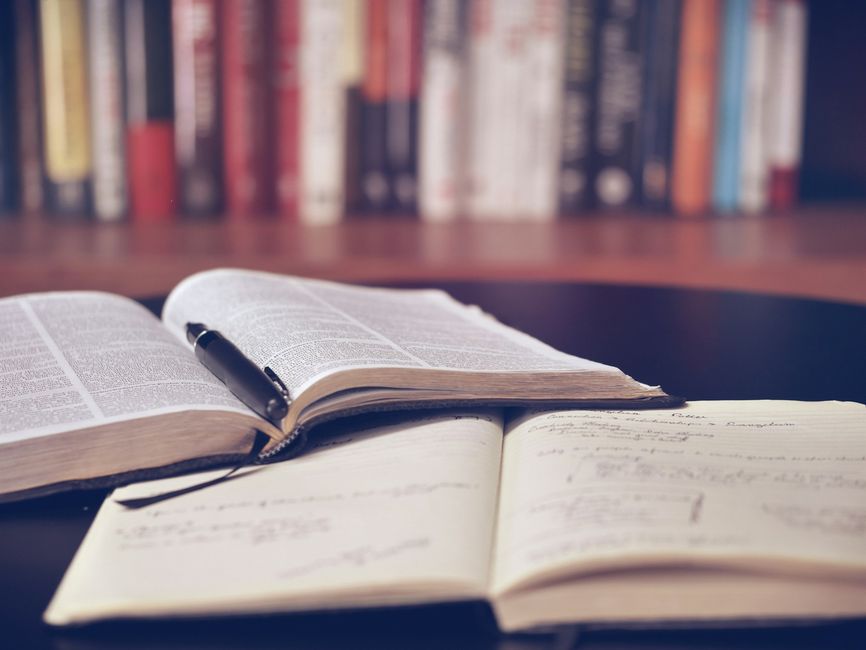
I recently encountered an interesting problem that changed what ID I'll give to the stacks in a CDK code.
What's the ID? Here's an example from the tutorial:
const app = new cdk.App();
new HelloCdkStack(app, 'HelloCdkStack', {
});
The ID here is HelloCdkStack
. The ID is important as by default the name of the stack is dependent on it. And since there can not be two stacks named the same deployed to the same region that usually implies that the same ID can not be deployed to the same region twice.
Originally, I did not give too much attention to the ID: I can't deploy twice to the same region, but that's usually not a requirement.
But then I needed to deploy the same stack in multiple regions in the same account and I was surprised to see it fail. Naively, I thought that since stacks are regional so two deployments with the same ID but to different regions should not interfere with each other.
This line of thinking works for regional resources: two Lambda functions in different regions can coexist no matter what. But there are resources that are global and those can introduce inter-stack conflicts.
The CDK provides a Names.uniqueId
function that generates a unique ID. It is useful when you need to provide a name: instead of hardcoding something that will result in a collision you can use this function that generates one for you.
The CloudFront PublicKey uses this function to generate a name:
private generateName(): string {
const name = Names.uniqueId(this);
if (name.length > 80) {
return name.substring(0, 40) + name.substring(name.length - 40);
}
return name;
}
How it works is that it walks the logical IDs of the components up until the root component and generates some hashes. This works inside a region as two stacks can not have the same name and the names are by default dependent on the stack ID, so the generated IDs will be different.
The problem here is that if the same stack with the same logical ID is deployed to multiple regions then this will generate the same name. And since the PublicKey is a global resource this will fail the deployment.
So the recommendation is to put the region into the stack's ID so that it can be safely deployed to multiple regions simultaneously:
const app = new cdk.App();
new HelloCdkStack(app, `HelloCdkStack-${process.env.AWS_REGION}`, {
});